How Developers Can Improve Their Debugging Techniques By Gustavo Woltmann
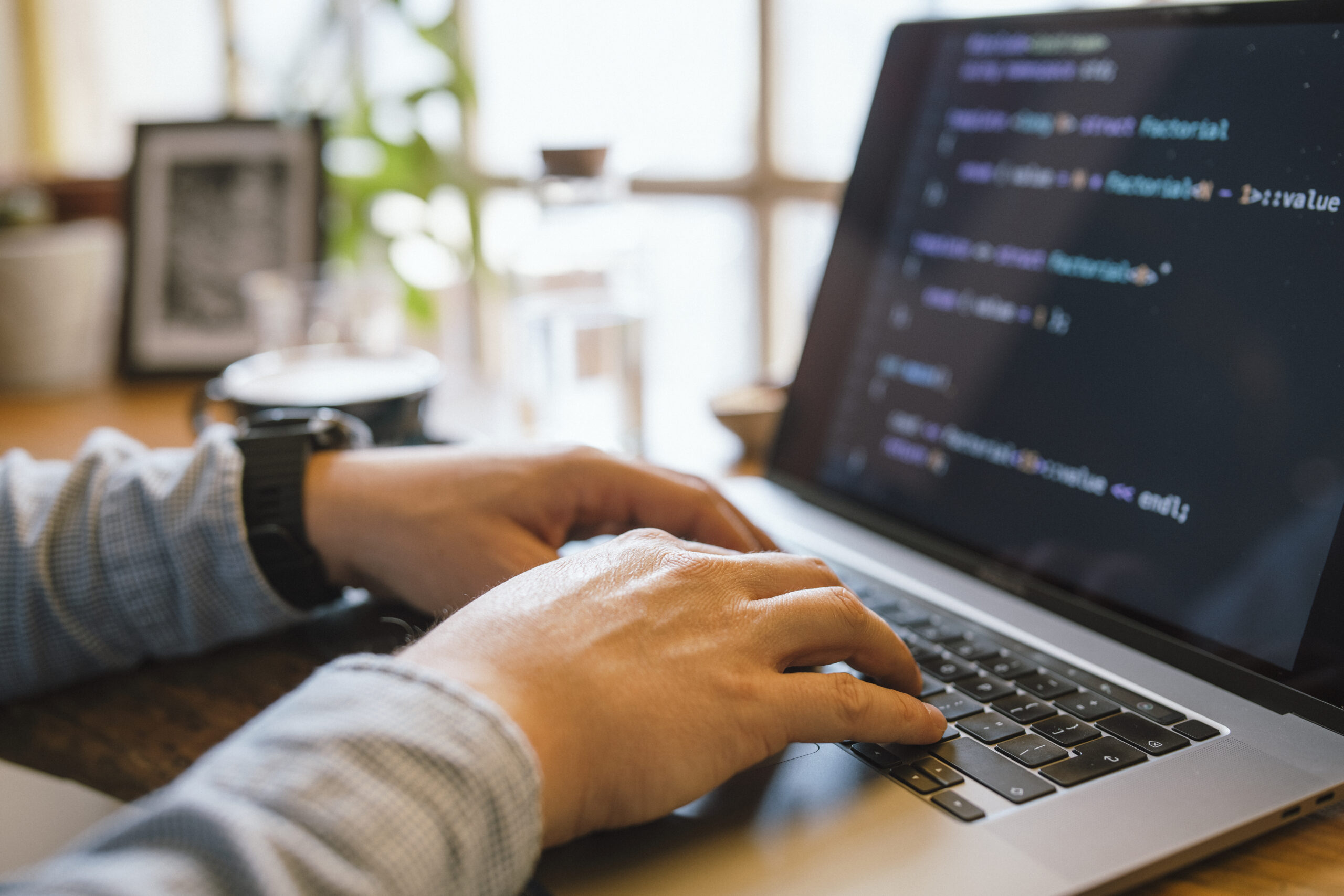
Debugging is Just about the most critical — however frequently neglected — techniques inside of a developer’s toolkit. It's not just about fixing damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Feel methodically to resolve complications competently. Whether you're a beginner or a seasoned developer, sharpening your debugging skills can preserve hrs of disappointment and substantially boost your productiveness. Listed below are various tactics to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Tools
One of the fastest strategies developers can elevate their debugging abilities is by mastering the tools they use everyday. Whilst writing code is a person Portion of enhancement, figuring out the way to connect with it properly throughout execution is Similarly significant. Modern day advancement environments arrive equipped with highly effective debugging capabilities — but many builders only scratch the surface of what these resources can perform.
Get, for instance, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, action by means of code line by line, and perhaps modify code over the fly. When utilised correctly, they Enable you to notice just how your code behaves throughout execution, which happens to be a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-end developers. They permit you to inspect the DOM, watch network requests, look at real-time functionality metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can change discouraging UI problems into workable responsibilities.
For backend or method-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Management around operating procedures and memory management. Finding out these applications might have a steeper Finding out curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be relaxed with Model Command systems like Git to comprehend code historical past, find the exact second bugs have been launched, and isolate problematic improvements.
Finally, mastering your applications implies heading outside of default options and shortcuts — it’s about producing an personal expertise in your improvement atmosphere in order that when concerns come up, you’re not dropped at nighttime. The higher you are aware of your applications, the greater time you could expend resolving the particular dilemma rather than fumbling as a result of the procedure.
Reproduce the condition
One of the more significant — and infrequently neglected — techniques in productive debugging is reproducing the challenge. Ahead of jumping into the code or making guesses, builders have to have to produce a regular surroundings or scenario where by the bug reliably seems. Without having reproducibility, repairing a bug turns into a game of prospect, generally resulting in squandered time and fragile code improvements.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask issues like: What actions resulted in The difficulty? Which setting was it in — advancement, staging, or output? Are there any logs, screenshots, or mistake messages? The greater depth you've got, the easier it will become to isolate the exact ailments below which the bug takes place.
After you’ve gathered more than enough data, try to recreate the challenge in your local setting. This could indicate inputting the identical details, simulating equivalent person interactions, or mimicking program states. If The difficulty appears intermittently, take into account creating automatic checks that replicate the edge scenarios or state transitions concerned. These assessments not just enable expose the issue and also prevent regressions Later on.
From time to time, The difficulty might be environment-certain — it would materialize only on particular working devices, browsers, or less than distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t merely a move — it’s a mindset. It needs persistence, observation, plus a methodical tactic. But as you can constantly recreate the bug, you happen to be by now halfway to fixing it. Using a reproducible situation, You need to use your debugging instruments much more efficiently, examination likely fixes safely and securely, and talk a lot more Obviously using your staff or buyers. It turns an summary grievance into a concrete problem — and that’s where developers thrive.
Read and Understand the Mistake Messages
Mistake messages in many cases are the most worthy clues a developer has when a thing goes Completely wrong. Rather then observing them as annoying interruptions, developers ought to learn to take care of mistake messages as immediate communications through the program. They frequently show you what precisely took place, in which it happened, and occasionally even why it transpired — if you know the way to interpret them.
Commence by studying the information meticulously and in comprehensive. Many builders, especially when less than time strain, glance at the 1st line and quickly begin creating assumptions. But further inside the mistake stack or logs may possibly lie the accurate root induce. Don’t just copy and paste mistake messages into search engines — examine and realize them to start with.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or perhaps a logic mistake? Will it level to a selected file and line amount? What module or functionality induced it? These thoughts can guidebook your investigation and stage you towards the liable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically stick to predictable designs, and learning to recognize these can considerably speed up your debugging approach.
Some faults are vague or generic, As well as in those circumstances, it’s very important to examine the context during which the mistake happened. Check connected log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede more substantial challenges and provide hints about likely bugs.
Finally, mistake messages are not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, lower debugging time, and turn into a extra economical and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When used effectively, it provides real-time insights into how an application behaves, helping you understand what’s happening under the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with understanding what to log and at what level. Common logging concentrations consist of DEBUG, Information, WARN, Mistake, and Deadly. Use DEBUG for thorough diagnostic details in the course of advancement, INFO for basic occasions (like successful start-ups), Alert for likely concerns that don’t break the applying, Mistake for genuine troubles, and FATAL in the event the process can’t keep on.
Steer clear of flooding your logs with excessive or irrelevant facts. Excessive logging can obscure significant messages and slow down your system. Center on essential occasions, point out alterations, input/output values, and significant selection factors in your code.
Structure your log messages clearly and continuously. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Permit you to monitor how variables evolve, what ailments are satisfied, and what branches of logic are executed—all with out halting This system. They’re especially worthwhile in production environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a effectively-assumed-out logging method, you may lessen the time it will require to identify problems, achieve further visibility into your purposes, and improve the All round maintainability and trustworthiness within your code.
Believe Similar to a Detective
Debugging is not just a technical activity—it is a method of investigation. To effectively recognize and correct bugs, builders will have to strategy the method similar to a detective resolving a secret. This mindset assists break down intricate difficulties into workable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or efficiency troubles. The same as a detective surveys against the law scene, accumulate just as much appropriate data as you may devoid of leaping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Upcoming, variety hypotheses. Check with oneself: What could possibly be leading to this conduct? Have any modifications lately been produced to the codebase? Has this issue happened in advance of less than identical situation? The target is usually to slim down prospects and determine potential culprits.
Then, test your theories systematically. Seek to recreate the condition inside of a managed surroundings. In the event you suspect a specific purpose or element, isolate it and verify if The problem persists. Like a detective conducting interviews, talk to your code thoughts and Permit the results guide you closer to the reality.
Shell out close notice to modest particulars. Bugs normally disguise inside the least predicted places—just like a missing semicolon, an off-by-just one error, or maybe a race situation. Be complete and affected individual, resisting the urge to patch the issue without the need of totally being familiar with it. Short term fixes may conceal the actual issue, just for it to resurface later.
And lastly, maintain notes on Anything you attempted and acquired. Just as detectives log their investigations, documenting your debugging course of action can conserve time for long run issues and aid Many others fully grasp your reasoning.
By thinking just like a detective, builders can sharpen their analytical skills, technique complications methodically, and turn out to be simpler at uncovering concealed problems in advanced units.
Compose Checks
Writing exams is one of the simplest tips on how to enhance your debugging capabilities and Over-all enhancement efficiency. Assessments not simply assistance catch bugs early but additionally serve as a safety Internet that provides you self esteem when earning variations to your codebase. A properly-examined software is simpler to debug as it means that you can pinpoint accurately where by and when a dilemma takes place.
Get started with device assessments, which focus on individual capabilities or modules. These compact, isolated checks can immediately expose irrespective of whether a selected bit of logic is Performing as predicted. Each time a take a look at fails, you promptly know the place to seem, drastically lowering the time spent debugging. Device assessments are Specifically helpful for catching regression bugs—problems that reappear after previously being preset.
Following, integrate integration tests and close-to-conclude exams into your workflow. These help make sure several areas of your application function alongside one another website efficiently. They’re especially practical for catching bugs that come about in sophisticated systems with many elements or services interacting. If a thing breaks, your exams can tell you which Element of the pipeline failed and less than what problems.
Writing assessments also forces you to Assume critically about your code. To check a feature thoroughly, you will need to know its inputs, envisioned outputs, and edge instances. This standard of comprehending The natural way prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong first step. After the exam fails regularly, you may focus on repairing the bug and enjoy your test pass when The problem is fixed. This method makes sure that the same bug doesn’t return Later on.
Briefly, crafting tests turns debugging from a annoying guessing activity into a structured and predictable method—supporting you capture extra bugs, quicker and even more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to be immersed in the situation—gazing your screen for hours, attempting Remedy soon after Option. But One of the more underrated debugging tools is simply stepping away. Using breaks aids you reset your brain, lessen stress, and sometimes see The problem from a new viewpoint.
When you're also close to the code for as well extended, cognitive tiredness sets in. You could possibly start off overlooking evident problems or misreading code that you just wrote just hrs earlier. Within this state, your Mind results in being a lot less successful at dilemma-fixing. A brief walk, a coffee crack, or maybe switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report discovering the foundation of a challenge once they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially through more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not merely unproductive but also draining. Stepping away means that you can return with renewed Vitality and a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
In the event you’re trapped, an excellent general guideline is usually to established a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to move all over, stretch, or do anything unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, but it really in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, using breaks will not be a sign of weak point—it’s a sensible strategy. It provides your Mind space to breathe, enhances your point of view, and helps you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a mental puzzle, and rest is a component of fixing it.
Master From Each and every Bug
Just about every bug you encounter is more than just A brief setback—It is really an opportunity to improve as a developer. Regardless of whether it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can educate you anything precious if you make an effort to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of critical thoughts as soon as the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Create more robust coding practices relocating forward.
Documenting bugs may also be a great behavior. Maintain a developer journal or preserve a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring challenges or prevalent issues—you could proactively prevent.
In crew environments, sharing Whatever you've discovered from the bug with the peers may be especially impressive. No matter if it’s by way of a Slack message, a brief create-up, or A fast expertise-sharing session, aiding others avoid the similar concern boosts team performance and cultivates a more powerful Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a few of the most effective developers are usually not those who write best code, but those who repeatedly learn from their problems.
In the end, Each and every bug you take care of adds a different layer for your ability established. So subsequent time you squash a bug, take a instant to reflect—you’ll arrive absent a smarter, more capable developer because of it.
Conclusion
Increasing your debugging skills will take time, observe, and patience — nevertheless the payoff is big. It makes you a more productive, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at That which you do.